Change the value of a variable (JS)
var variableCalculee;
execution.setVariable("nomDeLaVariableCible", variableCalculee);
Testing the existence of a variable
If a variable does not yet exist, when its value is called, it returns the following text : {id_variable_inexistante}
For example, in the case of a ternary condition to display or not the value of a variable when we do not know its existence.
// ${condition ? valeur_si_vrai : valeur si faux}
${variable_a_tester == "{variable_a_tester}" ? "La variable n'existe pas" : 'La variable existe et a pour valeur : '.concat(variable_a_tester)}
// En javascript
var variableAMettre = "nok";
if(typeof variableATester != "undefined"){
variableAMettre = variableATester;
}
execution.setVariable("maVariable", variableAMettre);
Calculate a date based on an original date and a deadline
var dateRecup = start_date;
var delaiRecup = start_delai;
var SEMAINE = 604800000;
var JOUR = 86400000;
var dateOK = new Date(dateRecup.getTime() - delaiRecup*JOUR);
execution.setVariable("dateCalculee", dateOK.toISOString());
Keeping a valuable history using JSON
In the case of a looping task, it may be necessary to keep some data that will be reset and therefore deleted:
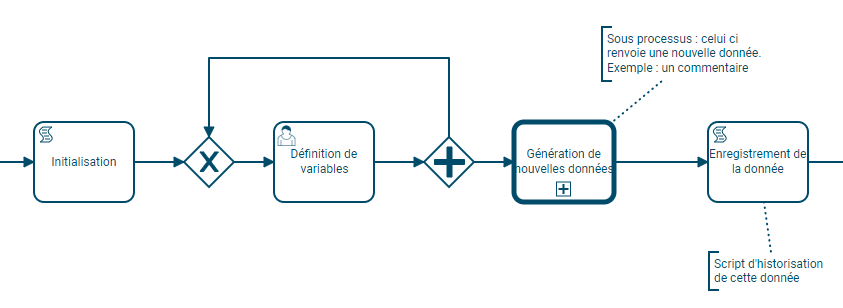
Example: The potential output data of the subprocess call should be retained. You then have to go through a script task that will add in a Json array each new value coming out of the sub-process call task.
This process is done in two steps:
- Initialization of the history variable
You have to initialize the variable that will be used next:
var arrayInitialization = [];
var json = JSON.stringify(arrayInitialization);
execution.setVariable("myVariableId_json", json);
- Recording new data
In the output of the task “Generation of new data”, in the following script task, we want to keep three variables: a comment variable and the email of the person who responded.
These two variables have as ID respectively: response_comment_out and response_email_out.
Here is the JS script to add these two new values to our Json variable:
// Récupération en JS de deux données provenant du processus
var js_comment = response_comment_out;
var js_email = response_email_out;
// Récupération du tableau précédemment initialisé
var tab = JSON.parse(myVariableId_json);
// Création d'une ligne qui comprend nos deux données à conserver
var newRow = {};
row.comment=js_comment;
row.email=js_email;
// Insertion de la ligne
tab.push(row);
// Ré-enregistrement du json
execution.setVariable("myVariableId_json", JSON.stringify(tab));
Find a next day of the week
In javascript, to recover for example the next Wednesday, whether in 1 or 6 days :
// Choix du jour de la semaine prochain.
// 0 pour dimanche, 6 pour samedi.
//Exemple ici avec mercredi
var indexOfNextDayOfWeek = 3;
// La date à partir de maintenant, on peut prendre n'importe quelle date
var date = new Date();
var currentDay = date.getDay();
var offset = indexOfNextDayOfWeek - currentDay;
// Deux cas de figures. Si on est mercredi, et qu'on souhaite quand même prendre
// le mercredi de la semaine prochaine, utiliser cette condition suivante
if (offset <= 0 ){
offset = offset +7;
}
// Sinon, si on veut conserver ce mercredi la, il faut utiliser "if (offset < 0 )"
// Calcul du prochain mercredi :
var nextWednesday = new Date(date.setDate(date.getDate() + offset ));
Formatting a number: setting the number of digits
It is possible to display a number with X required digits. For example, the numbers 2, 45 or 255 can be set to take the format 0002, 0045, 0255.
// Exemple avec 4 digits
var nbr = my_variable_iterop;
var nbr4digits = (nbr).toPrecision(4).split('.').reverse().join('');
// Autre méthode (toujours avec 4 digits)
var nbr = my_variable_iterop;
var nbr4digits = ("0000" + nbr).slice(-4);
Replace characters with others
Sometimes it can be useful to replace one string with another.
An example: the output of a multiple value in Iterop contains a ## as separator. If this data is to be used for example as a multiple e-mail recipient, then these ## must be replaced by commas. Here are the lines of code to use:
// La source
var sourceMails = "adresse1@mail.com##adresse2@mail.com##adresse3@mail.com";
// JS avec REGEX
var multiRecipients = sourceMails.replace(/##/g, ','));
// JS sans REGEX
var multiRecipients = sourceMails.split("##").join( ",");
// JUEL
${execution.setVariable("multiRecipients", sourceMails.replace("##", ",")};
Saving an array type object using Json
It is not possible to keep an Array object, created from a JS script using the split() method for example, in the Iterop system, because it is not serializable (the marketing department has not validated this term).
However, it is possible using Json to store the information in text form, and then find this same table in another script task for example.
// Création ou récupération d'un tableau dans myVar :
var myTab = otherVar.split('##');
// Stockage de l'information sous format JSON :
var myTabInString = JSON.stringify(myTab);
execution.setVariable("my_var_to_record", myTabInString)
// Récupération de la donnée au format tableau :
var tab = JSON.parse(myTabInString);
var myElement = tab[0];
JS/JUEL – Test the existence of a variable
If a variable does not yet exist, when its value is called, it returns the following text : {id_variable_inexistante}
For example, in the case of a ternary condition to display or not the value of a variable when we do not know its existence.
// JUEL
// ${condition ? valeur_si_vrai : valeur si faux}
${variable_a_tester == "{variable_a_tester}" ? "La variable n'existe pas" : 'La variable existe et a pour valeur : '.concat(variable_a_tester)}
// En javascript
var variableAMettre = "nok";
if(typeof variableATester != "undefined"){
variableAMettre = variableATester;
}
execution.setVariable("maVariable", variableAMettre);
JUEL – Add supervisors on a particular instance
It is possible to add supervisors/controllers for a specific instance during the execution of a process by means of a script task.
The script task will have to be configured in JUEL and can retrieve the value of a group, user or multi-user variable.
// Code Juel pour modifier les utilisateurs
// Ajoute les utilisateurs en superviseurs depuis la variable user.
${roles.addSupervisorUsers(variable_user_or_multiuser_id, execution)}
// Met à jour les superviseurs en fonction de la variable user.
// Voir Explications ci-après.
${roles.updateSupervisorUsers(variable_user_or_multiuser_id, execution)}
// Supprime les superviseurs ou contrôleurs depuis la variable user.
${roles.removeSupervisorUsers(variable_user_or_multiuser_id, execution)}
// Code Juel pour modifier les groupes (même fonction que pour les utilisateurs)
${roles.addSupervisorGroups(variable_gorup_or_multiGroup_id, execution)}
${roles.updateSupervisorGroups(variable_gorup_or_multiGroup_id, execution)}
${roles.removeSupervisorGroups(variable_gorup_or_multiGroup_id, execution)}
The function “updateSupervisorUsers” and “updateSupervisorGroups” allows to directly update all the supervisors from the value of a variable passed in parameter.
For example:
- In the variable, the value entered is User1, User2 and User3.
- User2 and User 4 are already execution supervisors
When passing through the script task, User2 will not be modified, User1 and User3 will be added, and User4 will be removed from the supervision.
General information on JUEL
JUEL is a language derived from java used in Iterop’s scripting tasks for example. Here’s how to declare a JUEL instruction:
${instruction Juel à saisir}
Most of the time, it is to call the value of a variable filled in a process :
${taskName_variableId}
This code will then return the value of the variable whose id is “taskName_variableId”.
The advantage of this language is that it can be embedded in certain strings (outside of a script task) such as in the name of a task for example :
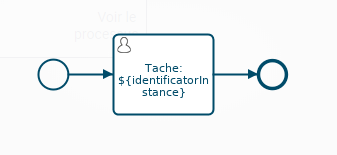
which gives in my tasks, by filling in the instance identifier “Send Quotation” :
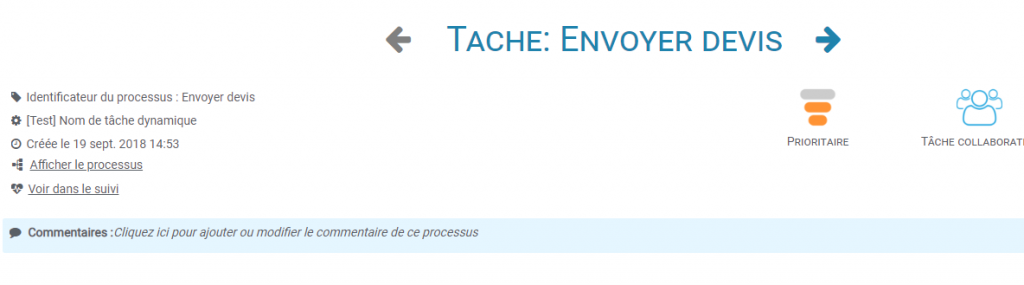
⚠ If a JUEL statement points to a variable that doesn’t exist (a wrong ID or the data was never entered or requested in the process), then the value returned will be the same id surrounded by braces.
For example:
Donnée existante : ${task_dataFromHumanTask}
// Cela affichera : Donnée existante : Ma donnée
Donnée non existante : ${task_unknownData}
// Cela affichera : Donnée non existante : {task_unknownData}
It is possible in JUEL to call up specific methods. These methods are described in the following pages of this documentation.
Calculate the time between two activities
You want to know the time lapse between the completion of two activities.
Indeed, it is important to understand why your process sometimes drags on and to be able to identify where the slowdowns are. It may be useful to display the time spent between the validation of two tasks.
This data can be calculated from KPIs but it is possible to retrieve it in a variable to be displayed in the monitoring table (and reporting) or to be used in the rest of the process (in an email for example).
The goal will be to use two scripting tasks.
- A task that will record the date and time of the start of the counter. This will be placed before the very first activity of the period you want to measure.
- The second script task will this time record the date and time of the last activity.
Here’s a simple representation:

The “Waiting” task represents the activity(ies) we want to time.
The “Calculation” task stops the counter and calculates the time spent between the two script tasks.
The “Visualization” task will just be used to block the process and display the counter.
First script task “Start”
var js_startDateCompteur = new Date();
execution.setVariable("script_startDateCompteur", js_startDateCompteur);
Second script task “Computation”
var js_startDateString = execution.getVariable("script_startDateCompteur");
if (js_startDateString !== null) {
var js_startDate = new Date(js_startDateString);
var js_endDate = new Date();
// Calcul de la durée en jours
// On soustraie le temps passé pour arriver jusqu'à la fin du compteur
// avec le temps passé pour arriver jusqu'au démarrage de ce compteur.
var js_differenceJour = (endDate.getTime() - startDate.getTime()) / 1000
/ 3600 / 24 ;
// Il est également possible de convertir cette durée en heure par
// exemple avec (endDate.getTime() - startDate.getTime()) / 1000
/ 3600 ;
execution.setVariable("script_differenceJour", js_differenceJour);
}
Reminder: the method getTime() of JavaScript returns a duration in milliseconds, so it is necessary to convert it (to day in this example).